How to debug Control Panel Applets in Pocket PC 2003 and Pocket PC 5.0 devices?
Recently while developing my En2FaMail control panel program i came into this problem.
Reading MSDN documentations and searching Internet,you will find you have to use ctlpnl.exe as your host program.But this doesn't seem to work.
Anyway before beginning,a short introduction to Control Panel Applets!
Control Panel Applets are basically dll files but with extension of cpl.And they have to export a function like this.
LONG CALLBACK CPlApplet (HWND hwndCPL, UINT uMsg, LONG lParam1, LONG lParam2)
And windows send following messages to communicate with this applet
CPL_GETCOUNT
CPL_INIT
CPL_NEWINQUIRE
CPL_STOP
There is also a sample in PocketPc 5.0(and i guess also PocketPc 2003) SDKS,name MyBacklight.
Also you can read following pages for more information on how to develop your own Control Panel Applets.
An Ancient Story of Control Panel Applets
Using Control Panel
So how to debug your program?
As Control Panel Applets are dll files they need a host,so to function and also for you to debug them.
I find out you can use mstli.exe as your host application.You have to set \windows\mstli.exe as your host in visual studio debugging page.
But if mstli.exe was ran for any reasons,first you have to kill this task.Eighter by visual studio or your own task manager in your PocketPc.(You cant use default memory control panel applet to kill this task,as it is not shown there).
After deploying and running,you also have to manually start your program,by going into settings and tapping on your application name.
Also it seems you have to do it not very slowly,as visual studio again terminates the task.
And then you are good to go!
Thursday, August 30, 2007
Saturday, August 25, 2007
Uniscribe and Delphi!
Microsoft has an extremely powerful API called Uniscribe that allows applications to do typography of scripts.Things that are mostly in interest of those who wants to make their programs multilanguage.
ut, one big problem with Uniscribe is the lack of samples and documentations over internet.
Problem :
I have a routine in my En2Fa library that gives me a widestring.If this WideString is displayed in right to left TLabel or TEditBox(i.e., in Delphi a component with BIDI set to right-to-left),you can see that it is displayed correctly.(You wont see the difference until you have both English and Persian-in my case-or both RTL and LTR texts in your string).
But if you display it in a window that doesn't have RTL attribute, some swapping of words will be noticed.
I wanted to use Uniscribe to fix this problem.
But why?
Well in my Y!En2FaMsngr program, I have to output this text into Yahoo Messenger editbox, and reading it correctly is not also an issue, but sending it correctly is more important.
So i have to reorder the text that it both displays ok and also sends to other party in correct way.
Making Yahoo Messenger edit box right to left is not a problem. You can use this code to make edit boxes right to left.
Searching over internet, and also looking at Uniscribe documentation in your SDK you will notice there are several functions that will do the job of displaying texts, but what I need is a logical form of reordered string. So I can use normal WM_SETTEXT to set text of yahoo EditBox.
Logical order is an order that you typed your strings. But visual order is how text should be displayed.
Look here how logical and visual orders are different.
So, I created ApplyRightToLeft function that tries to reorder logical string so it can be displayed ok in left to right EditBox.
To start you need Uniscribe Delphi unit files. You can download it from here.
Two important functions I used are ScriptItemize and ScriptLayout.
Look at the code below.
The job of ScriptItemize is to break a Unicode string into individually shapeable items and giving you SCRIPT_ITEM structures representing the items that have been processed.
Look at the result of ScriptItemize with following text.
And here is how it is formed in logical order.
So in my function generated_items will be 3.
After that things are easy, you have to calculate width of each run, and using ScriptLayout that gives you Logical to Visual table-which gives you an array showing which runs should be displayed first-.And showing it in reverse order is all you have to do.
Here are links that can make life a little bit easier when using Uniscribe.
Uniscribe
Unicode Script Processor for Complex Scripts
Supporting Multilanguage Text Layout and Complex Scripts with Windows NT 5.0
Design and Implementation of a Win32 Text Editor
Displaying Text with Uniscribe
Globalization Step-by-Step
Limitations of Uniscribe
Supporting multilanguage text layout and complex scripts with Windows 2000
Yes I know, I hate VB but...just for those interested
Tutorial Using Unicode in Visual Basic 6.0
ut, one big problem with Uniscribe is the lack of samples and documentations over internet.
Problem :
I have a routine in my En2Fa library that gives me a widestring.If this WideString is displayed in right to left TLabel or TEditBox(i.e., in Delphi a component with BIDI set to right-to-left),you can see that it is displayed correctly.(You wont see the difference until you have both English and Persian-in my case-or both RTL and LTR texts in your string).
But if you display it in a window that doesn't have RTL attribute, some swapping of words will be noticed.
I wanted to use Uniscribe to fix this problem.
But why?
Well in my Y!En2FaMsngr program, I have to output this text into Yahoo Messenger editbox, and reading it correctly is not also an issue, but sending it correctly is more important.
So i have to reorder the text that it both displays ok and also sends to other party in correct way.
Making Yahoo Messenger edit box right to left is not a problem. You can use this code to make edit boxes right to left.
lAlgn := GetWindowLong(hYahooEditHnd, GWL_EXSTYLE) ;
// To toggle alignment and reading order
lAlgn := lAlgn or WS_EX_RIGHT or WS_EX_RTLREADING;
SetWindowLong(hYahooEditHnd, GWL_EXSTYLE, lAlgn );
InvalidateRect(hYahooEditHnd, nil, false);
Searching over internet, and also looking at Uniscribe documentation in your SDK you will notice there are several functions that will do the job of displaying texts, but what I need is a logical form of reordered string. So I can use normal WM_SETTEXT to set text of yahoo EditBox.
Logical order is an order that you typed your strings. But visual order is how text should be displayed.
Look here how logical and visual orders are different.

To start you need Uniscribe Delphi unit files. You can download it from here.
Two important functions I used are ScriptItemize and ScriptLayout.
Look at the code below.
function ApplyRightToLeft(inText:WideString) : WideString;
type
SCRIPT_ITEMArray = array of SCRIPT_ITEM;
var
items:SCRIPT_ITEMArray;
control : SCRIPT_CONTROL;
state : SCRIPT_STATE;
generated_items,max_items : integer;
hr : HRESULT;
text_len,i : integer;
bidiLevel:array of byte;
LogicalToVisual ,VisualToLogical :array of integer;
widths:array of integer;
begin
Result := '';
text_len := Length(inText);
if text_len = 0 then
exit;
// Most applications won’t need to set any control flags.
ZeroMemory(@control, sizeof(SCRIPT_CONTROL));
// Initial state, you will probably want to keep this updated as you process
// runs in order so that you can always give it the correct direction of the
// surrounding text.
ZeroMemory(@state, sizeof(SCRIPT_STATE));
state.uBidiLevel := 0; // 0 means that the surrounding text is left-to-right.
max_items := 16;
while (true) do
begin
// Make enough room for the output.
SetLength(items,max_items);
// We subtract one from max_items to work around a buffer overflow on some
// older versions of Windows.
generated_items := 0;
hr := ScriptItemize(PWideChar(inText), text_len, max_items - 1, @control,
@state, @items[0], @generated_items);
if (SUCCEEDED(hr)) then
begin
// It generated some items, so resize the array. Note that we add
// one to account for the magic last item.
SetLength(items,generated_items + 1);
break;
end
else
if (hr <> E_OUTOFMEMORY) then
begin
// Some kind of error.
exit;
end;
// The input array isn't big enough, double and loop again.
max_items := max_items*2;
end;
SetLength(bidiLevel,generated_items);
SetLength(VisualToLogical ,generated_items);
SetLength(LogicalToVisual ,generated_items);
// Manually extract bidi-embedding-levels ready for ScriptLayout
for i := 0 to generated_items-1 do
bidiLevel[i] := items[i].a.s.uBidiLevel;
// Build a visual-to-logical mapping order
ScriptLayout(generated_items, @bidiLevel[0], @VisualToLogical [0],@LogicalToVisual [0]);
SetLength(widths,high(items)+1);
for I := 0 to high(items) do
items[i].iCharPos := items[i].iCharPos + 1;
for I := 0 to high(items) - 1 do
widths[i]:=items[i+1].iCharPos - items[i].iCharPos;
widths[high(items) ]:=1;
for I := generated_items - 1 downto 0 do
Result:=Result+copy(inText,items[VisualToLogical[i]].iCharPos,
widths[VisualToLogical[i]]);
// free the temporary BYTE[] buffer
bidiLevel := nil;
VisualToLogical := nil;
LogicalToVisual := nil;
widths := nil;
items := nil;
end;
The job of ScriptItemize is to break a Unicode string into individually shapeable items and giving you SCRIPT_ITEM structures representing the items that have been processed.
Look at the result of ScriptItemize with following text.

After that things are easy, you have to calculate width of each run, and using ScriptLayout that gives you Logical to Visual table-which gives you an array showing which runs should be displayed first-.And showing it in reverse order is all you have to do.
Here are links that can make life a little bit easier when using Uniscribe.
Uniscribe
Unicode Script Processor for Complex Scripts
Supporting Multilanguage Text Layout and Complex Scripts with Windows NT 5.0
Design and Implementation of a Win32 Text Editor
Displaying Text with Uniscribe
Globalization Step-by-Step
Limitations of Uniscribe
Supporting multilanguage text layout and complex scripts with Windows 2000
Yes I know, I hate VB but...just for those interested
Tutorial Using Unicode in Visual Basic 6.0
Wednesday, August 22, 2007
Intresting Site.
Well,i was just wondering how could i play midi files on PocketPcs.
So far it seems to be a complicated process,Or i didn't quite get it.
I am using FMODCE for my application,but unfortunately it doesn't support midi files.Long ago i used SDL + timidity for my Duke Nukem game to play midi files.
But well i really don't like the idea of shipping 30mg instrument files with my application.Seems the ArcSoft hast it,but not for free!
Anyway i ran into this interesting site,with a little bit strange,long name.
http://www.ifihadadollarforeverytimesomeonesaidthatiwouldhavemyownwebsite.com
So far it seems to be a complicated process,Or i didn't quite get it.
I am using FMODCE for my application,but unfortunately it doesn't support midi files.Long ago i used SDL + timidity for my Duke Nukem game to play midi files.
But well i really don't like the idea of shipping 30mg instrument files with my application.Seems the ArcSoft hast it,but not for free!
Anyway i ran into this interesting site,with a little bit strange,long name.
http://www.ifihadadollarforeverytimesomeonesaidthatiwouldhavemyownwebsite.com
Blogger filtered?
Well,but why only first page of it,and login page is censored?
Also comments are censored too.
It is really a pain to post in blogger.You have to use tor and login,then you can normally do the rest.
I think maybe Iranian weblog providers prefer to use theirs instead of Google,i see no other reason for blocking main page of blogger!
Also comments are censored too.
It is really a pain to post in blogger.You have to use tor and login,then you can normally do the rest.
I think maybe Iranian weblog providers prefer to use theirs instead of Google,i see no other reason for blocking main page of blogger!
Monday, August 20, 2007
Have you ever used Lazarus for Pocket PC development?
Well,i really don't know why still people are not -that much- using Lazarus + FPC for Pocket PC development.
I think the main problem is,it is not well advertised,and lots of people don't know about it yet.
Another factor could be that it is an open source software,and well people sometimes have some wrong ideas about open source software in general,like being unstable,not good looking and so on.
Anyway,i really think the best option for object pascal programmers is Lazarus + FPC for Pocket Pc development.
I really think it is also best choice for other developers trying to develop in win32.Don't you really hate C strings?MFC?ATL?
I know c/c++ really well,but being a long time turbo pascal and afterwards Delphi user,i really couldn't get the idea behind MFC and ATL.
It just don't work for me.
Here is list of my cons and pros.
Pros :
1- It feels like a really big,full featured,stable IDE environment and just looks like good Delphi 7.
2- Your programs will be based on LCL widget set which you can whenever you want simply migrate your program to Win32/Linux/Mac OS environments.
3- It is pascal/Delphi language.If you are familiar with VCL and Delphi.Everything here is the same.
4- You also have a good debugger.So debugging your programs in your retail device or also in emulators is piece of cake.
5- Lots and Lots of components and sources are available in Internet,you simply need to compile them for ARM/Pocket PC target.
6- Results are in native machine instructions,not in slow .net or java platforms.And no need that users installed these into their machines.
Cons
1- .exe files are bigger in comparison to what visual studio produces.But you can always safely use UPX or even switch into using KOL for your widgetset based programs.(look at kol-ce for sources).
Just that.I really see no other problem with current implementation of Lazarus and FPC.
Not to mention that my En2FaMail program is also compiled with FPC.based on my long time ago En2Fa program sources.
At first i tried to use Delphi 2005 .net cf implementations.
but when i just saw that much of errors on you can not use pointers and...
I abandoned the project.
Anyway,here is the screenshot i created when i was implementing LCL for wince just to convince anybody that everything was working.
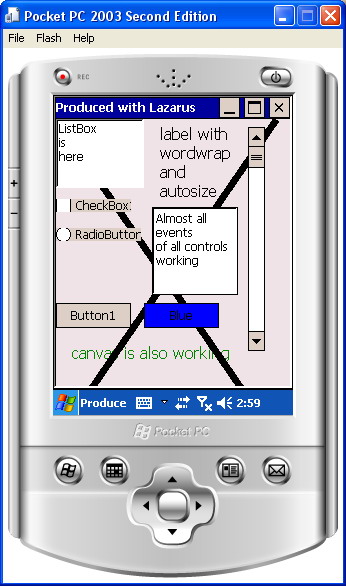
And LCL has improved a lot since then.
I hope i convinced some of you in how great could be development with LCL.Especially for those who are still using pure c for their programs,and those who hate .net!!
For more information please look at these URLs :
http://wiki.lazarus.freepascal.org/Windows_CE_Interface
http://wiki.lazarus.freepascal.org/Windows_CE_Development_Notes
http://wiki.freepascal.org/WinCE_port
I think the main problem is,it is not well advertised,and lots of people don't know about it yet.
Another factor could be that it is an open source software,and well people sometimes have some wrong ideas about open source software in general,like being unstable,not good looking and so on.
Anyway,i really think the best option for object pascal programmers is Lazarus + FPC for Pocket Pc development.
I really think it is also best choice for other developers trying to develop in win32.Don't you really hate C strings?MFC?ATL?
I know c/c++ really well,but being a long time turbo pascal and afterwards Delphi user,i really couldn't get the idea behind MFC and ATL.
It just don't work for me.
Here is list of my cons and pros.
Pros :
1- It feels like a really big,full featured,stable IDE environment and just looks like good Delphi 7.
2- Your programs will be based on LCL widget set which you can whenever you want simply migrate your program to Win32/Linux/Mac OS environments.
3- It is pascal/Delphi language.If you are familiar with VCL and Delphi.Everything here is the same.
4- You also have a good debugger.So debugging your programs in your retail device or also in emulators is piece of cake.
5- Lots and Lots of components and sources are available in Internet,you simply need to compile them for ARM/Pocket PC target.
6- Results are in native machine instructions,not in slow .net or java platforms.And no need that users installed these into their machines.
Cons
1- .exe files are bigger in comparison to what visual studio produces.But you can always safely use UPX or even switch into using KOL for your widgetset based programs.(look at kol-ce for sources).
Just that.I really see no other problem with current implementation of Lazarus and FPC.
Not to mention that my En2FaMail program is also compiled with FPC.based on my long time ago En2Fa program sources.
At first i tried to use Delphi 2005 .net cf implementations.
but when i just saw that much of errors on you can not use pointers and...
I abandoned the project.
Anyway,here is the screenshot i created when i was implementing LCL for wince just to convince anybody that everything was working.
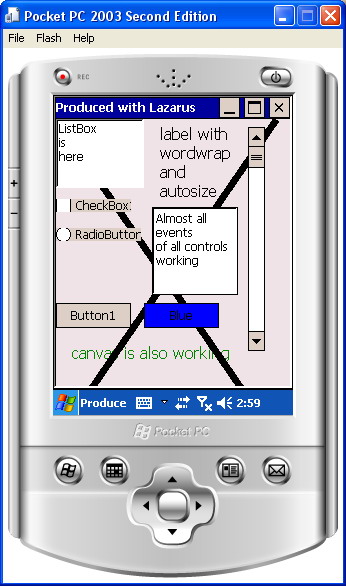
And LCL has improved a lot since then.
I hope i convinced some of you in how great could be development with LCL.Especially for those who are still using pure c for their programs,and those who hate .net!!
For more information please look at these URLs :
http://wiki.lazarus.freepascal.org/Windows_CE_Interface
http://wiki.lazarus.freepascal.org/Windows_CE_Development_Notes
http://wiki.freepascal.org/WinCE_port
Y!En2FaMsngr Program or (Yahoo Messenger + En2Fa) or How not use fingilish in Yahoo Messenger program
Sometimes i wonder,when all this En2Fa*s will end.
I guess NEVER.
Anyway,this is again my En2Fa program for your Yahoo Messenger.
This program convert/translate all your incoming messages(which are mostly in fingilish - pinglish) into Persian.
Very easy to use.
Also no problem if your messages are too in Persian,program simple ignores them and displays them OK.
You can grab the program from here.
From programming side of things,I simply injected a dll into Yahoo Messenger,and also some html injection to,and not to forget that I've had to create an Activex module too.
It really took sometime from me,but worth it.
Hope i can discuss it with you soon.
Sunday, August 19, 2007
En2Fa

Well,i know it might be old.The program i mean.
But becouse i lost my site,maybe putting it againg here in my blog will help peaple using google search to find a software that translate/convert fingilish/pingilish/pengilish words or sentences into very good and readable persian/farsi.
You can find always find out more with some googling.
I bet you wont find anything better than this software,besides it is only 500kb.Worth downloading.
Here is my En2Fa program.
Saturday, August 18, 2007
my En2FaMail program.

This is my En2Fa program for Pocket PC Phones.
You can grab it from here.
I think it is mostly for use of people living in Iran,But other Persian talking people can also benefit from this program.
As my main program,En2Fa this program Converts fingilish/pingilish typing into readable Persian language.
Pingilish or fingilish is kind of language,simply invented because of problems with no available Persian fonts or Persian keyboard.
You can find the definition from wikipedia like this (well actually i created that page :) ):
"Fingilish (Farsi + English) (also Penglish or Pinglish, Finglish, Pingilish and Fārgilisi) is a term used to describe the way Persian words are written in Latin alphabet. This type of writing is commonly used in online chat, emails and SMS."
So this program simply do the hard job of translating fingilish into persian.
But what is En2FaMail?
It translates your SMSs into Persian.And the interesting -and for me real hard-part is you really do not need to install any special font,or Arabic/Persian language program to being able to read Persian.
En2FaMail takes care of everything.
Also you can set special sounds in formats like .mp3,.ogg or .mod to your incoming SMSs.
Also side notes are that,this program can be easily disabled from control panel(settings) of your device.
And does not change your SMSs in inbox,simply displays them in Persian.
So feel free to use it and also recommend it to others.
Some features of this program :
- Changing your fingilish/pinglish SMSs into Persian/Farsi language.
- All settings can be changed from control panel(Pocket PC settings)
- No need for Persian/Arabic Making softwares,like Arabizer.
- You can view Persian incoming SMSs in systems without Persian font and Arabic/Persian making software.
- All original SMS features like,Save as read,reply,call sender is implemented.
- All SMS text is viewed in notification,for bigger SMSs scroll bars will appear so you can scroll and real your SMS
- Delivery reports are fixed.So there will be no more numbers in your delivery reports,but Contact names will be replaced.
- Persian Delivery report texts also exist.
- You can assign notification sounds in formats of .mp3,.mod,.mid,.ogg,.wav to your incoming SMSs
- And many more....
But hey this is a programming weblog,So
I will be starting my MAPI and some other lessons soon.All regarding this software.
Actually i find many interesting things to say.
Hope in this week or next week i can post something about Inbox Mail Rules and...
Thursday, August 16, 2007
How to promote this weblog?
I am relatively new in writing weblogs.
I was just imagining how can i get more visitors and peaple to come and read my weblog?
First i thought i have to be so good that just google search result will bring peaple here.
But i really feel the need of other peaple comments and support.
So i guess to go places and advertise.
Anyway,..
I was just imagining how can i get more visitors and peaple to come and read my weblog?
First i thought i have to be so good that just google search result will bring peaple here.
But i really feel the need of other peaple comments and support.
So i guess to go places and advertise.
Anyway,..
Tuesday, August 14, 2007
Lazarus made it easy!
After only spending 1 hour with lazarus to modify my very old delphi code,which was more than 20 forms and about 30 units,I finally ported this program into my Pocket PC.
Just 1 hour!!I myself couldn't imagine how easy it was to port a Delphi program into Lazarus.
Can you believe it?
Amazingly,it runs without any problems and also functioned so well.
Only some bugs in repainting.Menus were having issues too,some visual problems.
Of course i need to patch the codes in lazarus for menus.My last patch was a quick hack to make things just work.
Oh,it is been more than 4 months since my last commit into Lazarus CE Interface.I should find some time for it,i guess.
Just 1 hour!!I myself couldn't imagine how easy it was to port a Delphi program into Lazarus.
Can you believe it?
Amazingly,it runs without any problems and also functioned so well.
Only some bugs in repainting.Menus were having issues too,some visual problems.
Of course i need to patch the codes in lazarus for menus.My last patch was a quick hack to make things just work.
Oh,it is been more than 4 months since my last commit into Lazarus CE Interface.I should find some time for it,i guess.
Monday, August 13, 2007
Do you want to use Windows CE SDK 5.0 headers but compile for 2003 or previous versions?
Do you want to use Windows CE SDK 5.0 headers but compile for 2003 or previous versions?
Beside the answer,you might ask why would anybody want to do this?
Well,i ran into this case.
Compatibility and single binary file for different versions of pocketpcs.
If you want to run your .exe file in both PocketPc 5.0 and 2003 versions,the simplest solution is to compile it as Pocket PC 2003(ARMV4) in your Visual Studio.
But How can you use new definitions,defined in newer sdk files?
Copy/Paste?
I am really not a big fan of copy/paste.Just don have any good reasons to convince you,but I've had really bad problems with copy/pasting stuff,so i try as much as possible to prevent it.
Or,
Another reason is you want to compile for 2003 but debug it under 5.0 emulator.
or,
To simple do this.I don't know really another reasons,but i just wanted to do this.
Another reason is AFAIR you could do the same with embedded visual studios.
You only have to use under_ce definition to link to previous version of sdk(2002).
So here is the answer :
1. Set Buffer security check in c/c++ compiler tab in Project Options to no.
2. Set additional library directory in general/linker tab to your 2003 sdk lib directory.
mine is in "E:\Microsoft Visual Studio 8\SmartDevices\SDK\PocketPC2003\Lib\armv4"
3. Add /MACHINE:ARM to commands in linker.
4. I also changed _under_ce and win32_wce definitions in compiler tab into 0x420 .
Beside all this there are some things you should know when trying this approach.
Stay away from specific function in newer SDKs,or if you have to use LoadLibrary and GetProcAddress after checking for the right OS version.
Beside the answer,you might ask why would anybody want to do this?
Well,i ran into this case.
Compatibility and single binary file for different versions of pocketpcs.
If you want to run your .exe file in both PocketPc 5.0 and 2003 versions,the simplest solution is to compile it as Pocket PC 2003(ARMV4) in your Visual Studio.
But How can you use new definitions,defined in newer sdk files?
Copy/Paste?
I am really not a big fan of copy/paste.Just don have any good reasons to convince you,but I've had really bad problems with copy/pasting stuff,so i try as much as possible to prevent it.
Or,
Another reason is you want to compile for 2003 but debug it under 5.0 emulator.
or,
To simple do this.I don't know really another reasons,but i just wanted to do this.
Another reason is AFAIR you could do the same with embedded visual studios.
You only have to use under_ce definition to link to previous version of sdk(2002).
So here is the answer :
1. Set Buffer security check in c/c++ compiler tab in Project Options to no.
2. Set additional library directory in general/linker tab to your 2003 sdk lib directory.
mine is in "E:\Microsoft Visual Studio 8\SmartDevices\SDK\PocketPC2003\Lib\armv4"
3. Add /MACHINE:ARM to commands in linker.
4. I also changed _under_ce and win32_wce definitions in compiler tab into 0x420 .
Beside all this there are some things you should know when trying this approach.
Stay away from specific function in newer SDKs,or if you have to use LoadLibrary and GetProcAddress after checking for the right OS version.
Sunday, August 12, 2007
Windows Mobile - How to call inbox's compose form.
Well,i recently ran into this problem.How can i show inbox's compose form with some fields filled?
It seems to be fairly easy enough in .net cf but i am not interested at all in .net so i wanted to do this from c/c++.
Here is the answer :
You have 2 choices,if you want to target windows mobile or Windows CE 5 or 5.01 or higher you can use MailComposeMessage.
For older devices like 2003 edition,you have to try launching tmail.exe program with some arguments.
tmail.exe -service "SMS" -TO "myfriend" -SUBJECT "Hi" -BODY "Just to say hi"
It seems to be fairly easy enough in .net cf but i am not interested at all in .net so i wanted to do this from c/c++.
Here is the answer :
You have 2 choices,if you want to target windows mobile or Windows CE 5 or 5.01 or higher you can use MailComposeMessage.
For older devices like 2003 edition,you have to try launching tmail.exe program with some arguments.
tmail.exe -service "SMS" -TO "myfriend" -SUBJECT "Hi" -BODY "Just to say hi"
Friday, August 10, 2007
First post
Well,i think first post is always the hardest post.I haven't been around blogs and posting stuff for a while.
But recently i find out that there are lots of information i can share with,or at least before having hard time myself finding them, i hoped somebody had posted it.
So that's the reason i will be having this blog.
Well if i have to summarize my blog will mostly cover following areas.
- Windows Mobile and Windows CE
- Delphi/fpc/Lazarus programming
- Hope it also contain some engineering stuff around mathematics and civil engineering.
Well,i guess it is enough for first post.
But recently i find out that there are lots of information i can share with,or at least before having hard time myself finding them, i hoped somebody had posted it.
So that's the reason i will be having this blog.
Well if i have to summarize my blog will mostly cover following areas.
- Windows Mobile and Windows CE
- Delphi/fpc/Lazarus programming
- Hope it also contain some engineering stuff around mathematics and civil engineering.
Well,i guess it is enough for first post.
Subscribe to:
Posts (Atom)